Misc documentation for pyana.sara module¶
The idea of the page is a copy from my personal memo (in my Evernote).
Getting SwimPacket¶
from pyana.swim import SwimSciCount
import urllib
from Sadppac import *
bmu = SwimSciCount.getBmuUri(942)
fnam, inf=urllib.urlretrieve(bmu)
reader = SwimBmuReader()
reader.readFile(fnam)
sarray=reader.getArray()
sarray.getPacket(30)
This is a low-level way of getting Swim packet.
Getting CenaPacket¶
from pyana.util.pyanarc import RcFile
rc=RcFile()
baseuri=rc.get('cena', 'bmuobase')
uri='%s/orb_%04d/orb_%04d.cena'%(baseuri, orbnr, orbnr)
try:
local, flag = urllib.urlretrieve(uri)
except IOError, e:
return None
cbr=Sadppac.CenaBmuReader()
cbr.readFile(local)
carr = cbr.getArray()
This is a low-level way of getting CENA packet.
Getting SWIM observation time¶
Return the SWIM packet time. Returned is the array of the datetime.datetime
.
dat = pyana.swim.swim_start.getobstime(timerange=[t0, t1])
Getting SWIM packet (high level)¶
This supports only for the start counter, but really useful way of getting the count rate, flux, and distribution function of SWIM.
from pyana.swim import swim_start
import datetime
# To get science data (start counter) ve ry simple, refer to pyana.swim.swim_start module.
dat=swim_start.getvdf(datetime.datetime(2009,1,25,14,0,0), filter=[swim_start.filter.remove_one_count])
dat=swim_start.getdata(datetime.datetime(2009,1,25,14,0,0), filter=[swim_start.filter.remove_one_count])
dat=swim_start.getdeflux(datetime.datetime(2009,1,25,14,0,0), filter=[swim_start.filter.remove_one_count])
print dat.getData()
Returned is an instance of pyana.util.julday.JdObject
. You can access the data throught getData method.
Removing background of SWIM¶
There are several algorithms implemented. The swim_start.filter
module is the implementation.
One way is to run
pyana.swim.swim_start.getdata()
function with filter keyword.
If filters are specified, each filter is processed by order.
dat=swim_start.getvdf(datetime.datetime(2009,1,25,14,0,0), filter=[swim_start.filter.remove_background_5numsum, swim_start.filter.remove_one_count])
This is equivalent to the following code (for getdata() function)
swim=swim_start.getdata(datetime.datetime(2009,1,25,14,0,0)) # JdObject
swim16x16 = numpy.array(swim.getData()) # getData() returns 16x16 array.
swim16x16_nominal = numpy.array(filter.remove_background_5numsum(swim16x16))
swim16x16_nominal = numpy.array(filter.remove_one_count(swim16x16_nominal))
# Then convert to velocity distribution functions.
Energy of SWIM¶
import pyana.swim.SwimEnergy
etbl=pyana.swim.SwimEnergy.EnergyTable.table(version=2)
# etbl.getEnergy() # Central energy, but obsolete way.
etbl.getTable() # Central energy
etbl.getRange() # Energy range (2x16 array. Bounds are the average of the neighboring bins. To integral or to plot energy-time diagram, this method should be used.)
etbl.getFwhmRange() # Energy range with FWHM. Means that overwraps/gaps are allowed. For calibration or detailed analysis, this method should be used.
The illustration depicts the returned energy by getXXX() methods.
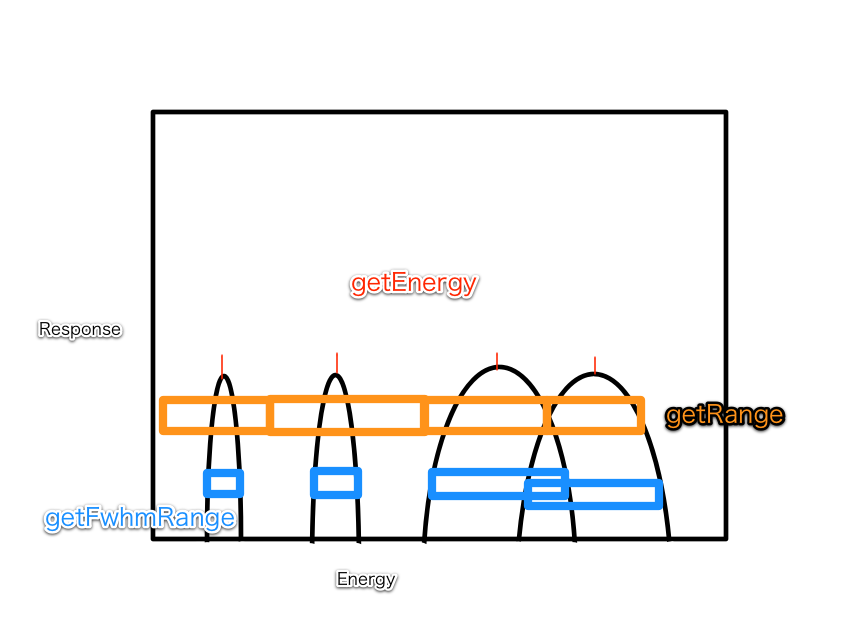
Energy of CENA¶
import pyana.cena.energy
etbl1 = pyana.cena.energy.getEnergy(1)
etbl2 = pyana.cena.energy.getEnergy(2)
etbl3 = pyana.cena.energy.getEnergy(3)
er1 = pyana.cena.energy.getRange(1)
efr3 = pyana.cena.energy.getFwhmRange(3)
There are three SV tables for CENA. You have to specify it.
Velocity vector of SWIM¶
To get SWIM velocity vector corresponding to 16x16 energy and directions, use
v=pyana.swim.swim_fov.velocity16x16sc()
v=pyana.swim.swim_fov.velocity16x16lse(datetime.datetime(2009,1,25,14,0,0))
Orbit number of CY-1¶
import pyana.cy1orb.Cy1OrbitNr
orbnr=pyana.cy1orb.Cy1OrbitNr.Cy1OrbitNr()
orbnr.setFromDefaultUri()
print orbnr.getStartTime(100) ## Returns the epoch time (float)
print orbnr.getStopTime(100)
print orbnr.getOrbitNr(datetime.datetime(2009,5,10,12,30,5))
Position of CY-1¶
pos = pyana.cy1orb.pvat2.getlsepos(datetime.datetime(2009,1,25,14,0,0))
Below is deprecated. Use pyana.cy1orb.pvat2
instead as above.
pos = pyana.cy1orb.pvat.getlsepos(datetime.datetime(2009,1,25,14,0,0))
To get the position in the ME frame, use pyana.cy1orb.pvat2.getmepos()
.
pos = pyana.cy1orb.pvat2.getmepos(datetime.datetime(2009,1,25,14,0,0))
In this case, a list [longitude, latitude, height] is returned.
Solar wind parameters observed by ACE¶
from pyana.swim.ace import AceMoon
import datetime
ace=AceMoon()
# ace.read(2009,1,25)
ace.getList(datetime.datetime(2009,1,25,13,0,0), datetime.datetime(2009,1,25,14,0,0))
lst=ace.getList(datetime.datetime(2009,1,25,13,0,0), datetime.datetime(2009,1,25,14,0,0))
for sw in lst:
print sw
print ace.getAverage(datetime.datetime(2009,1,25,13,0,0), datetime.datetime(2009,1,25,14,0,0))
If corresponding data is not available for some reasons, float(‘nan’) is filled for the data.
See pyana.swim.ace
module for details.
Conversion of the coordinate systems between the SC, LSE and ME.¶
### The conversion from the SC frame to LSE frame is done
### by pvat module. Conversion LSE to ME frame is done by
### lseme module.
### First, you have to instance Vector3d class such as
vec_sc = vector3d.Vector3d(1, 0.5, -0.5)
print 'Vector in SC = ', vec_sc.x, vec_sc.y, vec_sc.z
### Then, you can convert the vector to LSE frame.
t = datetime(2009,3,10,15,20,0) # conversion is time dependent.
vec_lse = pvat.getlsevec(t, vec_sc)
print 'Vector in LSE = ', vec_lse.x, vec_lse.y, vec_lse.z
### LSE to ME can be done by lse2me function.
### Note: lse2me takes array as an input and output.
vec_lse_arr = [vec_lse.x, vec_lse.y, vec_lse.z]
vec_me = lseme.lse2me(t, vec_lse_arr)
print 'Vector in ME = ', vec_me[0], vec_me[1], vec_me[2]
Conversion of the coordinate system between CENA and SC.¶
from pyana.cena import frame
frame.sc2cena(vec) # vec is either Vector3d or arraylike.
frame.cena2sc(vec)
Eclipse or not¶
rom pyana.cy1orb.eclipse import EclipseDat
e = EclipseDat()
e.inEclipse(datetime(2009, 1, 25, 15, 0, 0))
CENA FoV. Central value.¶
from pyana.cena.centralpixel import defaultDirsSc
dirs = defaultDirsSc()
# 7 element Vector3d array.
or
from pyana.cena.fov import *
theta = elev_pix_center(n)
phi = azim_pix_center(n)
from pyana.cena.frame import *
dir = angle2cena(theta, phi, degrees=True, vector3d=True)
It is not a good idea to have two methods for identical purposes. I think I need to take one of the in the near future.
So far, we run a test case to agree the results on (fovTest.py).
CENA observation time in mass mode¶
pyana.cena.cena_mass2.getobstime(timerange=None, orbit=None)
CENA mass mode data (high level)¶
from pyana.cena.cena_mass2 import *
dat = getdata(datetime.datetime(2009, 4, 18, 1, 30, 0)) # 8x7x128 numpy array
datE16 = getdataE16(datetime.datetime(2009, 4, 18, 1, 30, 0)) # 16x7x128 masked_array's JdObject.
Getting only hydrogen data, you can add up mass index 0 to 63.
print datE16[:, :, :64].sum(2)
CENA mass spectra plot¶
see the script script/plot_mass_spectra.py
Get Moon position in GSE¶
Use :mod:pyana.cy1orb.MoonPos
module.