SWIM mass analysis and one count event¶
SWIM is a mass analyzer, so we can know the mass information.
Getting raw data¶
Mass data is in the compressed part of SWIM packet.
libsadppac
will extract the packet, but now python analysis package, pyana.swim support
high-level functions on reading these count rate data.
Note that the physical unit conversion is not yet supported (2011-10-27).
Simplest way of getting count rate data is using a function
irfpy.swim.swim_mass.getdata()
.
>>> from irfpy.swim import swim_mass
>>> import datetime
>>> t = datetime.datetime(2009, 1, 25, 14, 0, 0)
>>> massdata = swim_mass.getdata(t)
>>> tobs = massdata.getDatetime()
>>> print tobs
2009-01-25 13:59:59.842
>>> cnts = massdata.getData()
>>> print cnts
[[[ 0., 0., 0., ..., 0., 0., 0.],
[ 0., 0., 0., ..., 0., 0., 0.],
[ 0., 0., 0., ..., 0., 0., 0.],
......
>>> print cnts.shape
(16, 16, 32)
As you may see in the above sample program, the irfpy.swim.swim_mass.getdata()
gets the time and returns the nearest data that has been observed.
Returned is the irfpy.util.julday.JdObject
instance.
The matrix (numpy.array
) has a shape of 16x16x32. The order is energy,
direction and mass channel.
For processing a large packet, you may better to use irfpy.swim.swim_mass.getobstime()
to get the real observation time. This will fasten the processing time significantly.
Below is an example.
>>> t0 = datetime.datetime(2009, 1, 25, 14, 0, 0)
>>> t1 = datetime.datetime(2009, 1, 25, 14, 20, 0)
>>> tlist = swim_mass.getobstime(timerange=[t0, t1]) # Obtain the exact time list of data.
>>> print tlist
(Julday(2009, 1, 25, 14, 0, 07.841), Julday(2009, 1, 25, 14, 0, 15.841), ....
>>> print len(tlist) # Number of SWIM data (packet) contained.
150
>>> for t in tlist: # Loop to process the observed data
... massdata = swim_mass.getdata(t) # Here JdObject is returned.
... # Some processing for massdata
Calibration¶
SARA development team in IRF has found a problem, so-called one count event, during the mission operation. I am not sure the source of the one count event, but if I remember correctly, this may be due to the crosstalk(?) from electronics (or TDC?) [- TBC with MW]. Anyway, some times the sensor took a specific event where no physical count is there. The pseudo count has (probably) always the same TOF, thus, the TOF channels should be removed before scientific analysis.
In the irfpy.swim.swim_mass
module, the reduction is implemented using “filter” functionality.
Filtering, in general, will mask or process the raw data set to convert to more calibrated data.
For one count event removal, there are four implementations according to the strength of the removal.
The implementation is in the irfpy.swim.swim_mass.filter.remove_one_count_evelt_l1()
(weakest) to
irfpy.swim.swim_mass.filter.remove_one_count_evelt_l4()
(strongest).
The function(s) can be taken as a filter
keyword in irfpy.swim.swim_mass.getdata()
method.
The way of using is as follows:
# Getting raw data
>>> t = datetime.datetime(2009, 1, 25, 14, 0, 0)
>>> l0data = swim_mass.getdata(t).getData()
>>> print l0data.sum()
1538.0
# Getting L1 removal of one count event
>>> l1data = swim_mass.getdata(t, filter=[swim_mass.filter.remove_one_count_event_l1]).getData()
>>> print l1data.sum()
1528.0
# Getting L2 removal of one count event
>>> l2data = swim_mass.getdata(t, filter=[swim_mass.filter.remove_one_count_event_l2]).getData()
>>> print l2data.sum()
1353.0
# Getting L3 removal of one count event
>>> l3data = swim_mass.getdata(t, filter=[swim_mass.filter.remove_one_count_event_l3]).getData()
>>> print l3data.sum()
1244.0
# Getting L4 removal of one count event
>>> l4data = swim_mass.getdata(t, filter=[swim_mass.filter.remove_one_count_event_l4]).getData()
>>> print l4data.sum()
1062.0
Sample of calibration¶
There is a sample code at swim_mass_ene_spectra.py
.
% python swim_mass_ene_spectra.py 2009-06-24T00:00:00 2009-06-25T00:00:00
Resulting figures are as follows.
Raw data¶
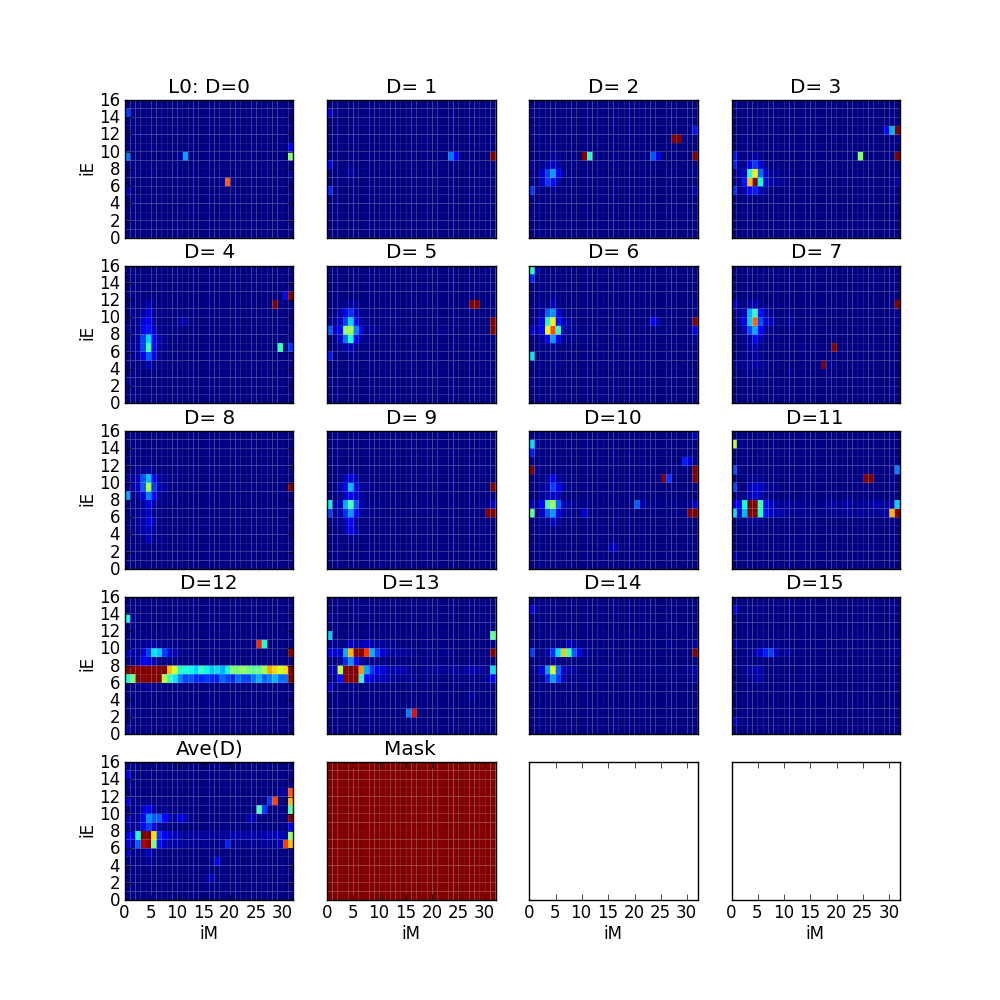
L1 data¶
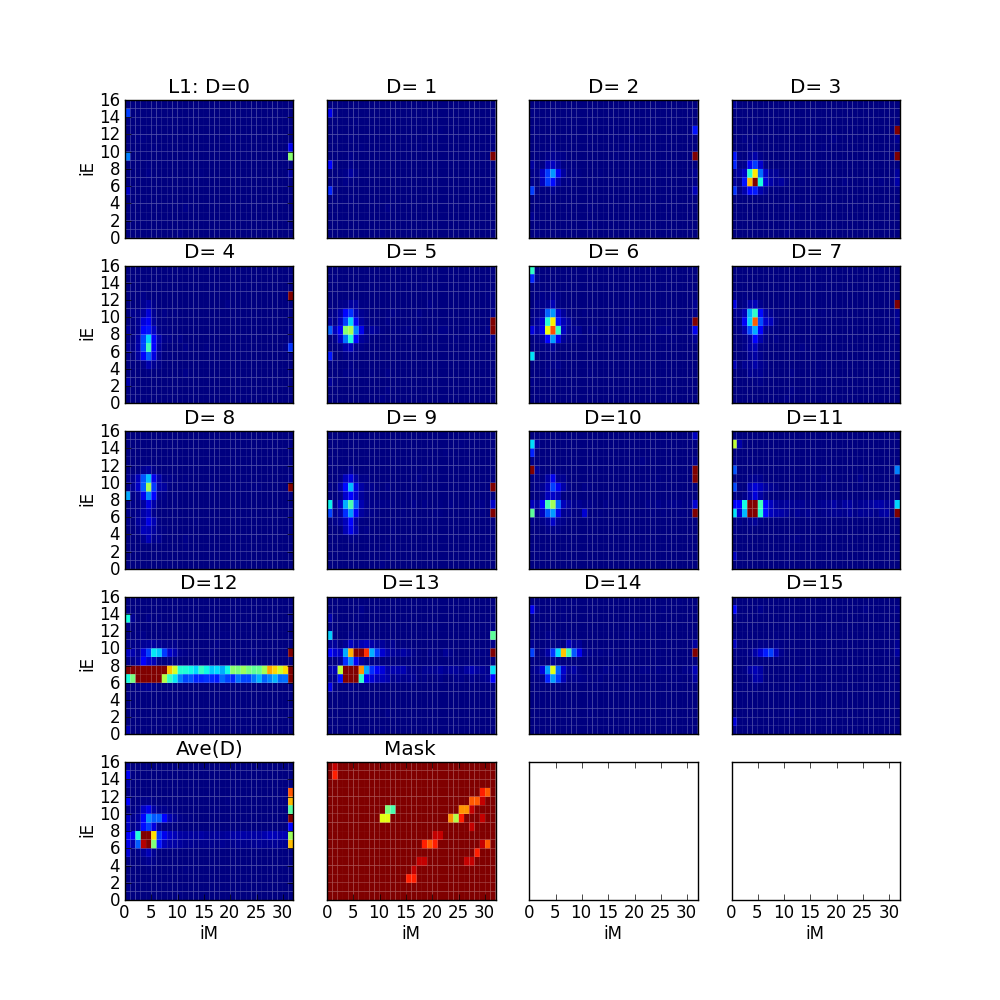
L2 data¶
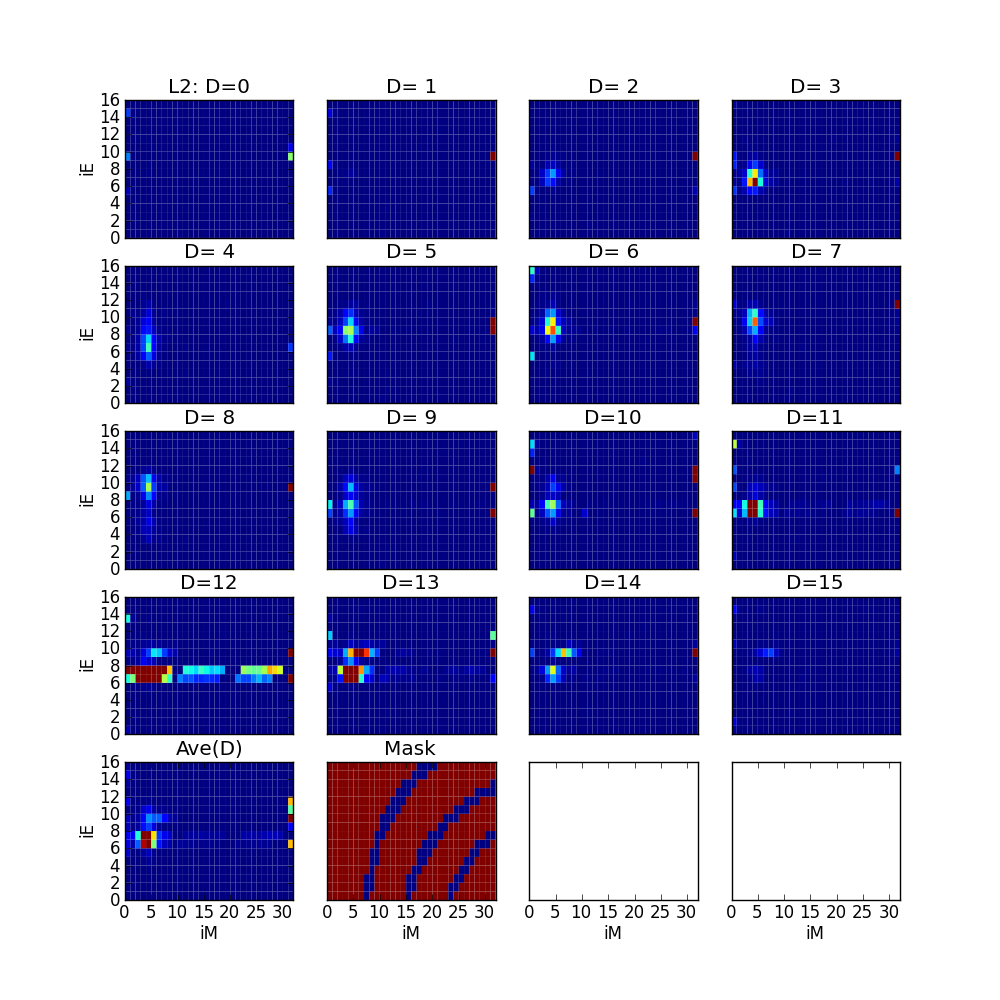
L3 data¶
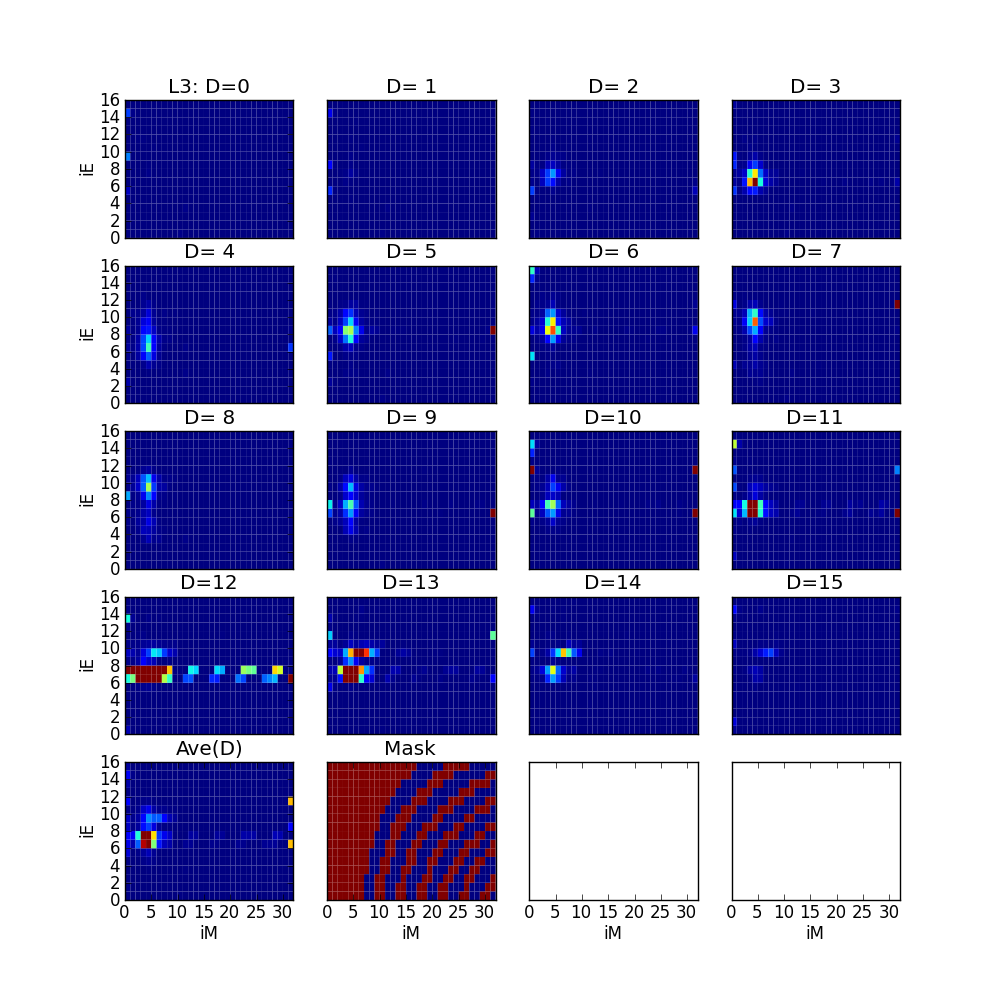
L4 data¶
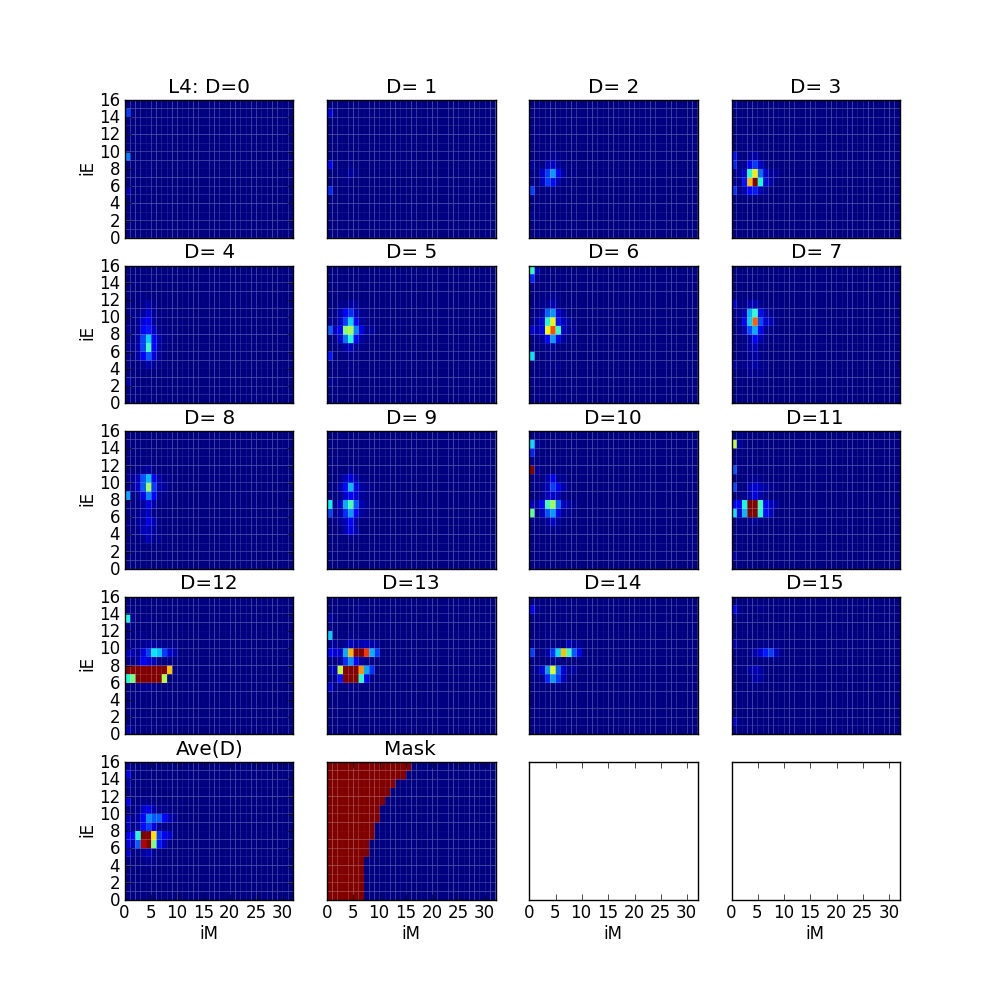