snippet_mars.exosphere_min
ΒΆ
A sample to plot Martian exosphere density profile for solar min.
The densities of the Martian exosphere are implemented in the module irfpy.mars.exosphere
.
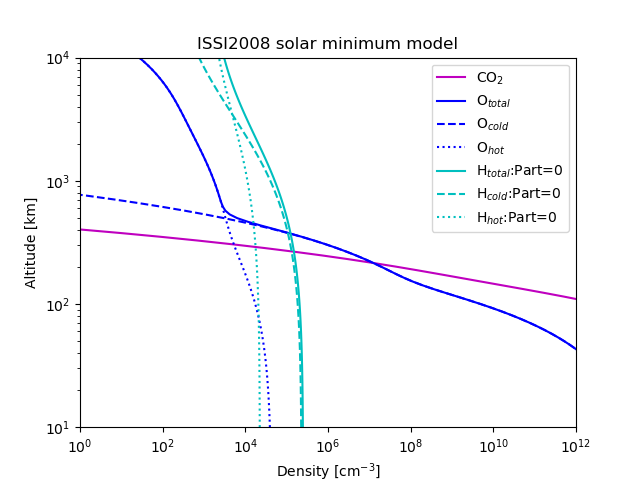
""" A sample to plot Martian exosphere density profile for solar min.
The densities of the Martian exosphere are implemented in the module :mod:`irfpy.mars.exosphere`.
.. image:: ../../../src/scripts/snippet_mars/ISSI2008_solmin.png
"""
import numpy as np
import matplotlib.pyplot as plt
from irfpy.mars.exosphere import ISSI2008_SolarMin as exosph_min
def main():
heights = np.logspace(1, 4, 100) # Heights from 10 km to 10000 km with 100 data points
# CO2 density
co2 = exosph_min.CO2(heights)
# O density
o_cold = exosph_min.O_cold(heights)
o_hot = exosph_min.O_hot(heights)
o_tot = o_cold + o_hot
# H denisty
part = 0 # Partition function. Default 0.
h_cold = exosph_min.H_cold(heights, part=part)
h_hot = exosph_min.H_hot(heights, part=part)
h_tot = h_cold + h_hot
# Plot
plt.subplot(111, xscale='log', yscale='log',
xlim=[1e0, 1e12], ylim=[1e1, 1e4],
xlabel='Density [cm$^{-3}$]', ylabel='Altitude [km]',
title='ISSI2008 solar minimum model')
plt.plot(co2, heights, color='m', label='CO$_2$')
plt.plot(o_tot, heights, color='b', label='O$_{total}$')
plt.plot(o_cold, heights, color='b', ls='dashed', label='O$_{cold}$')
plt.plot(o_hot, heights, color='b', ls='dotted', label='O$_{hot}$')
plt.plot(h_tot, heights, color='c', label='H$_{total}$:Part=0')
plt.plot(h_cold, heights, color='c', ls='dashed', label='H$_{cold}$:Part=0')
plt.plot(h_hot, heights, color='c', ls='dotted', label='H$_{hot}$:Part=0')
plt.legend()
plt.savefig('ISSI2008_solmin.png')
if __name__ == "__main__":
main()